Bot Execute
Invoke a Medplum Bot using the custom $execute
operation.
If you're not familiar with Medplum Bots, you may want to review Bot Basics documentation first.
Invoke a bot by ID
Invoke a bot by ID when you know the Bot's ID in advance.
Finding your Bot Id
You can find the id
of your Bot by clicking on the Details tab of the Bot resource. In this example, it is 43ac3060-ff20-49e8-9682-bf91ab3a5191
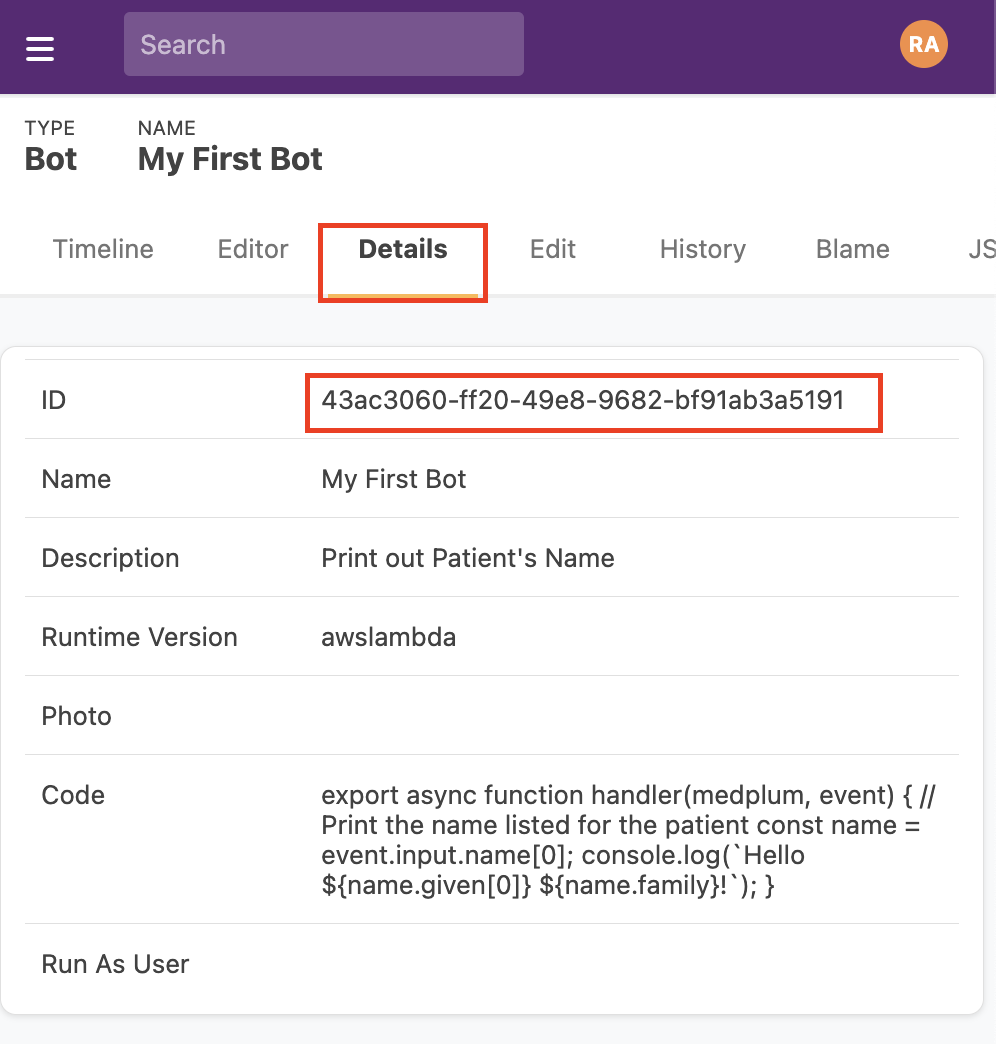
Using POST
POST [base]/Bot/[id]/$execute
Examples
- TypeScript
- CLI
- cURL
The MedplumClient TypeScript class provides a executeBot
convenience method
const result = await medplum.executeBot(id, { input: { foo: 'bar' } });
console.log(result);
medplum login
medplum post 'Bot/[id]/$execute' '{ "foo": "bar" }'
curl 'https://api.medplum.com/fhir/R4/Bot/[id]/$execute' \
-X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $MY_ACCESS_TOKEN" \
-d '{"foo":"bar"}'
Using GET
Query parameters will be passed to the bot as type Record<string, string>
(see Content Types below)
GET [base]/Bot/[id]/$execute?params
- TypeScript
- CLI
- cURL
const getResult = await medplum.get(medplum.fhirUrl('Bot', id, '$execute').toString() + '?foo=bar');
console.log(getResult);
medplum login
medplum get 'Bot/[id]/$execute?foo=bar'
curl 'https://api.medplum.com/fhir/R4/Bot/[id]/$execute?foo=bar' \
-H "Authorization: Bearer $MY_ACCESS_TOKEN"
Invoke a bot by identifier
Sometimes you may not know the Medplum Bot ID in advance. In that case, you can invoke a Bot by Identifier
.
This is also useful when the same conceptual bot exists in multiple Medplum projects. Each bot will have a different ID, but they can all have the same identifier.
Using POST
POST [base]/Bot/$execute?identifier=[system]|[code]
Examples
- TypeScript
- CLI
- cURL
The MedplumClient executeBot
convenience method supports both id: string
and identifier: Identifier
:
const result = await medplum.executeBot(
{
system: 'https://example.com/bots',
value: '1234',
},
{
foo: 'bar',
}
);
console.log(result);
medplum login
medplum post 'Bot/[id]/$execute?identifier=https://example.com/bots|1234' '{ "foo": "bar" }'
curl 'https://api.medplum.com/fhir/R4/Bot/$execute?identifier=https://example.com/bots|1234' \
-X POST \
-H "Content-Type: application/json" \
-H "Authorization: Bearer $MY_ACCESS_TOKEN" \
-d '{"foo":"bar"}'
Using GET
Query parameters will be passed to the bot as type Record<string, string>
(see Content Types below)
GET [base]/Bot/$execute?identifier=[system]|[code]¶ms
- TypeScript
- CLI
- cURL
const getResult = await medplum.get(
medplum.fhirUrl('Bot', '$execute').toString() + '?identifier=https://example.com/bots|1234&foo=bar'
);
console.log(getResult);
medplum login
medplum get 'Bot/$execute?identifier=https://example.com/bots|1234'
curl 'https://api.medplum.com/fhir/R4/Bot/$execute?identifier=https://example.com/bots|1234'\
-H "Authorization: Bearer $MY_ACCESS_TOKEN"
Content Types
Medplum Bots support a variety of input content types. Specify the input content type using the standard Content-Type
HTTP header, or as an optional parameter to MedplumClient.executeBot()
.
Content-Type | typeof event.input | Description |
---|---|---|
text/plain | string | <INPUT_DATA> is parsed as plaintext string |
application/json | Record<string, any> | <INPUT_DATA> is parsed as JSON-encoded object |
application/x-www-form-urlencoded | Record<string, string> | <INPUT_DATA> is parsed as URL-encoded string, resulting in a key/value map |
application/fhir+json | Resource | <INPUT_DATA> is parsed as a FHIR Resource encoded as JSON |
x-application/hl7-v2+er7 | HL7Message | <INPUT_DATA> is a string that should be parsed as a pipe-delimited HL7v2 message. HL7v2 is a common text-based message protocol used in legacy healthcare systems |
The input data that will be parsed according to CONTENT_TYPE
and passed into your Bot as event.input
.